I have come up with a technique to allow the user to edit content “Inline”.
Lets look at an example. I have a table of data, and the user needs to be able to edit the “Description” Field. Rather than take the user away from this ui view, I wish to enable “Inline Editing” like many powerful applications are offering these days.
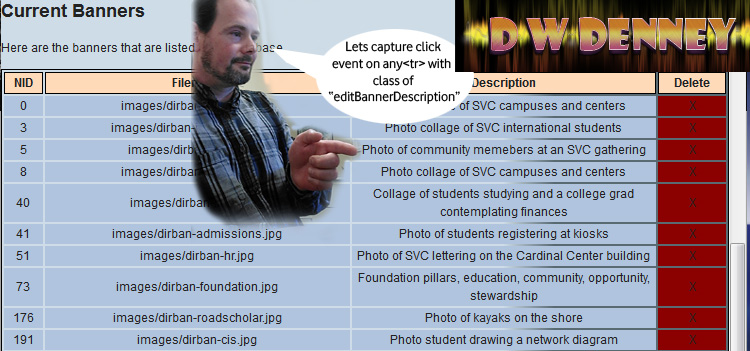
The first step is to assign functions to the correct fields and capture the users input events. The user would click on the field to select it, so lets set up a doc delegate event to capture a click on the description field. We need to use doc delegate because the data is dynamic and so isn’t in the DOM when the events handlers are normally assigned. Read my post about JQuery Document Delegate to catch up on this technique. I am going to delegate the capture of a click event on any table row <tr> with a class of “editBannerDescription”
$("#bannerslist").on("click", ".editBannerDescription", function(e){ var thedata=$(this).html(); alert(thedata); });
Yeah boyeee we have captured a click event for any element in the bannerslist table, and have access to the content that is in the table cell.
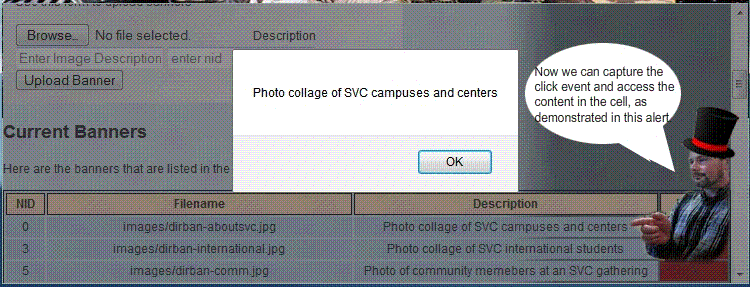
The next step is to alter the ui view so the user can edit the contents of the description table cell. I use a JQuery / JavaScript function to accomplish this:
enableEditDescription(object, bannernid){ console.log("Message from enable Edit Description function: lets enable to edit the description of slide id:"+bannernid+" from description of : "+$("#"+object).html()); }
I will continue to modify the enableEditDescription function to alter the ui view when the user clicks. I will grab the content from the cell, and replace the content with a text area whose value is the text that was in the cell.
The final code looks like this:
$("#bannerslist").on("click", ".editBannerDescription" , function(e){ var thedata=$(this).html(); var object=$(this).attr("id"); var getbannernid = object.match(/d+$/); if(getbannernid){ bannernid=parseInt(getbannernid[0], 10); console.log("Message from banner admin inc, here in doc delegate for editBannerDescription with content of :"+thedata+" in cell object id: "+object+" for banner nid: "+bannernid); enableEditDescription(object, bannernid);} else{ alert('error getting id');} }); // end #bannerslist doc delegate actions
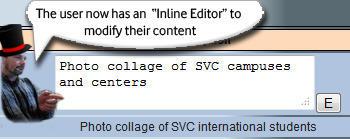
Now, when the user clicks a table cell with editable content, the cell changes into an inline editor.
The user needs to be able to “escape out” of the edit mode, discarding any changes that were made in the field.
Lets start with capturing the escape key press event when the user is in an editing field. We’ll use the good ol doc delegate to capture this as so…
$("body").on("keydown", "textarea, input", function(e){ if(e.keyCode==27){ console.log("We have key press of escape key, on event target " + e.target.id + " lets exit edit mode without saving changes. Lets decide what place they're editing...");} }); // end on keydown event handler
Now I can put back the original content of the cell and the user will have “escaped out” of edit mode.
The next step is going to be really fun. We are going to capture the press of the “Edit” button and save the new content into our cms.