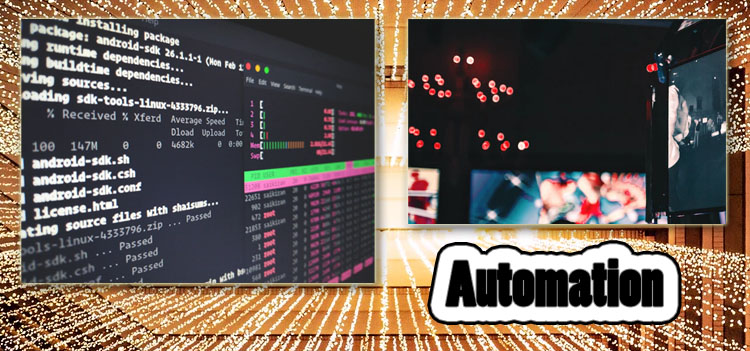
As complex paperless forms become full fledged web applications, there is a growing need for automation such as having a form available to be used from a certain date/time range. For example, a health insurance sign up that opens on December 1 at 9:00 AM and closes on December 24th at 5:00pm Pacific Time. We want these times to be hard coded for the server, in case the users are from different time zones, we want everyone to be able to sign up at exactly the same server time, regardless of their location. So if they were from New York, they would be open to sign up at 3pm December 1st, etc….
We need a few variables.
open_date – the date / time that the form will open
close_date – the date / time that the form will close
today_date_server – the current date/time of the servertoday_date_user – the current date/time of the users machine.
user_time_difference – the difference between the users time and the servers time. this could be used to display correct messages about when the user can sign up.
openorclosed – this will be set by the logic then used to display the correct messages and ui views
closedmessage – this will be a spot for the special message that will display to the user when the form is closed.
So now that I have my variables set up, lets put them to use. I will compare the dates and make sure that today is within the form opening range. Then I will set the openorclosed variable to open or closed based on the results. The I will update the ui view according to the openorclosed variable.
Here is the final form opening and closing automation logic in VbScript:
open_date = "9/2/" & year(NOW) & " 09:00 AM"
close_date = "10/14/" & year(NOW) & " 05:00 PM"
today_date_server = NOW()
datediffopen = DateDiff("n",today_date_server, open_date)
datediffclosed = DateDiff("n", today_date_server, close_date)
If datediffopen < 0 and datediffclosed > 0 Then
openorclosed = "open"
Else
openorclosed = "closed"
End If
This will automatically open and close the form to the users every year. Hooray for Automation!
Now I can manipulate the ui view using the variables, so the user will know when the form is open and why its closed.
[code language=”vb”]
If datediffopen > 0 and datediffclosed > 0 Then
‘display message for before the form has opened for the year.
openorclosedmessage=”The nomination form will be open from ” & open_date & ” to ” & close_date & “. Please submit your nominations between this time period for them to be considered.”
ElseIf datediffopen < 0 and datediffclosed < 0 Then
‘display message for after form has closed for the year.
openorclosedmessage=”The nominations were due by ” & close_date & “. The nominations are over for the ” & year(NOW) & ” year. The nomination form is closed.”
End If[/code]
To display the date and time in a user friendly format – Tuesday, October 14, 2014 at 5:00:00 PM – use the FormatDateTime function:
[code language=”vb”]FormatDateTime(close_date, 1) at FormatDateTime(close_date, 3)[/code]
To debug the automation code, use this snippet:
[code language=”vb”]
‘ this message is for de bugging the open / close automation
closedmessage = closedmessage + “<p class=’redtext boldtext’>Today is ” & today_date_server & “. The form will open on ” & open_date & ” and close on ” & close_date & “. Is today inside the date range?” & isitopenorclose & “. The date dif open is ” & datediffopen & “. the date diff closed is ” & datediffclosed & “</p>”
[/code]
Now I would like to take this a bit further, by having the form open on the first Monday of January, and close on the last Friday of January. Here is the algorithm I have come up with:
[code]
mydate = “1/1/” & year(NOW) & ” 9:00 AM”
dtmDate = CDate(mydate)
daycounter = 1
Do While daycounter <= 7
‘ response.write(Weekday(dtmDate) & ” is the weekday for ” & dtmDate & “<br/>”)
If Weekday(dtmDate) = 2 Then
response.write(“<br>The form will open on ” & FormatDateTime(dtmDate, 1))
Exit Do
End If
daycounter = daycounter + 1
dtmDate = dtmDate + 1
Loop
daycounter = 1
Do While daycounter <= 31
‘response.write(“<br />” & Weekday(dtmDate) & ” is the weekday for ” & dtmDate & “<br/>”)
If Weekday(dtmDate) = 6 Then
thelastfriday=dtmDate
End If
daycounter = daycounter + 1
dtmDate = dtmDate + 1
Loop
response.write(“<br>The form will close on ” & FormatDateTime(thelastfriday, 1))
[/code]
For the year 2014, the result will display:
The form will open on Monday, January 06, 2014
The form will close on Friday, January 31, 2014
Now I can drop this into my forms that will open in the pattern of first Monday, and close on last Friday of January.